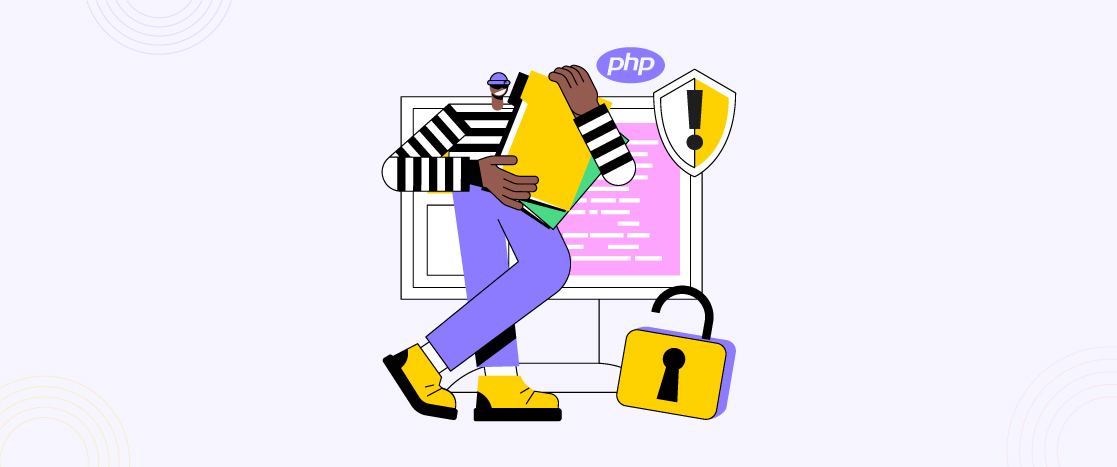
PHP Security
PHP is the most commonly used server-side programming language, powering over 80% of websites. It is behind many popular content management systems (CMS) such as WordPress, Joomla, Magento, and Drupal. Because of its widespread use, PHP is often a target for cyberattacks. Therefore, developers must prioritize PHP security to protect sensitive data and keep web applications safe from common vulnerabilities. In this article, we will look at the security issues that affect PHP applications and share best practices to protect them effectively.
Common Security Issues in PHP
PHP applications are vulnerable to several security issues, some due to the language, while others come from poor coding practices. These vulnerabilities allow attackers to exploit the application, access unauthorized data, and expose sensitive information. Below are some common security risks in PHP applications:
- Cross-Site Scripting (XSS): This happens when attackers inject malicious scripts into a web application executed in the user’s browser. These scripts can steal sensitive data like login credentials or session cookies.
- SQL Injection: In this attack, attackers manipulate SQL queries to access or alter data in the application’s database. If user input isn’t adequately validated, attackers can run harmful SQL commands, leading to unauthorized access or data breaches.
- Cross-Site Request Forgery (CSRF): This attack tricks authenticated users into performing unintended actions, like changing account settings or transferring funds. It takes advantage of the web application’s trust in the user’s browser.
- Session Hijacking: In this type of attack, an attacker steals session cookies or IDs to impersonate a legitimate user. This gives the attacker access to the user’s account, allowing them to act like the original user.
- Insecure File Uploads: When a PHP application allows file uploads, improper validation can let attackers upload files containing harmful scripts or malware. These files can then be executed on the server, compromising the system.
By understanding these vulnerabilities and taking steps to address them, developers can improve the security of their PHP applications and protect them from potential attacks.
How Secure is PHP?
PHP has been criticized in the past due to security vulnerabilities. However, over the years, it has dramatically improved security. With continuous updates and the developing of more secure frameworks, PHP has become better at defending against common threats. Modern versions of PHP, especially PHP 7 and 8, have introduced several significant improvements that fix past vulnerabilities and boost overall performance.
- PHP 7.x and PHP 8.x: These versions bring key security upgrades, such as better password hashing algorithms, improved error handling, and enhanced memory management. These updates make PHP more secure than earlier versions. For example, PHP 7 introduced the password_hash() function, which makes secure password storage more manageable, and PHP 8 improved performance and error handling to reduce the risk of attacks.
Upgrading to the latest stable version of PHP is crucial for reducing security risks and ensuring your applications are protected from known vulnerabilities.
Although no programming language is entirely free from security flaws, PHP can be just as secure as other popular server-side languages like Python, Java, or Ruby. This is especially true when developers follow secure coding practices and use modern tools and frameworks. Developers can build strong, secure applications protected from cyberattacks by keeping up with PHP’s latest versions and implementing best security practices.
PHP Security Best Practices
Here are some best practices for PHP security you must follow as a developer.
Update PHP Regularly
Regularly updating PHP is one of the most essential steps in securing your web application. Each new version of PHP includes important security fixes and performance improvements that protect your app from known vulnerabilities. Older versions of PHP, like PHP 5.x and PHP 7.0, no longer receive official support or updates, making them more vulnerable to attacks.
It’s crucial to upgrade to at least PHP 7.4 or PHP 8 to take advantage of the latest security features and improve performance. These newer versions offer stronger security, such as improved password hashing, better error handling, and protection against common threats.
When upgrading to a newer version, ensure your code is compatible with the updates. Tools like the PHP Compatibility Checker can help you find deprecated features in your code that may not be supported in newer versions. This lets you fix issues before upgrading, ensuring a smooth transition without breaking your application.
Cross-Site Scripting (XSS)
Cross-site scripting (XSS) attacks happen when attackers inject malicious JavaScript or other harmful code into a web page in a user’s browser. These attacks can steal sensitive information, change page content, or even perform actions on behalf of the user without their consent. XSS attacks are more dangerous because they exploit users’ trust in a website.
The best approach to preventing XSS attacks is to validate and clean all user input before showing it on your web pages. This ensures that any harmful code is neutralized before it can run in the user’s browser.
A simple and effective way to prevent XSS attacks in PHP is by using the htmlspecialchars() function. This function converts special characters (like <, >, &, and “) into HTML entities. Doing this will display any malicious script injected into the input as plain text that has not been executed.
Here’s an example of how to use htmlspecialchars() to secure user input in PHP:
$search = htmlspecialchars($_GET[‘search’], ENT_QUOTES, ‘UTF-8’);
echo “Search results for ” . $search;
In this example:
- $_GET[‘search’] retrieves the user input from the URL query parameter.
- htmlspecialchars() converts special characters in the input into HTML entities.
- ENT_QUOTES ensures both double and single quotes are encoded.
- ‘UTF-8’ specifies the character encoding to handle non-ASCII characters correctly.
Using this method, any dangerous code, such as <script> tags or other HTML, becomes harmless text displayed on the page instead of running in the browser. This is a simple but effective way to protect your application from XSS attacks.
SQL Injection Attacks
SQL injection is one of the most dangerous threats to PHP applications. It happens when user input is directly added to SQL queries, allowing attackers to manipulate the query and run harmful commands on the database. This can lead to unauthorized access, theft, or destruction.
The best way to protect your application from SQL injection is to use prepared statements with PDO (PHP Data Objects). Prepared statements separate the SQL code from the user input, treating the input as data rather than executable code. This prevents attackers from injecting harmful SQL into your queries.
Here’s an example of how to use prepared statements in PHP with PDO:
$sql = “SELECT username, email FROM users WHERE id = :id”;
$stmt = $dbh->prepare($sql);
$stmt->execute([‘:id’ => $id]);
In this example:
- $sql defines an SQL query with a placeholder (:id) to represent user input securely.
- $dbh->prepare($sql) prepares the query, making it ready to be executed.
- $stmt->execute([‘:id’ => $id]) safely binds the user input ($id) to the placeholder and runs the query. The input is treated as data, not part of the SQL code, so it won’t be executed even if the user tries to inject harmful SQL.
PHP uses prepared statements to ensure user input cannot interfere with the SQL query structure, effectively preventing SQL injection attacks. This simple but powerful method protects your application from one of the most common and harmful types of attacks.
Cross-Site Request Forgery (XSRF/CSRF)
Cross-Site Request Forgery (CSRF) attacks seriously threaten web applications. In a CSRF attack, an attacker tricks an authenticated user into performing unwanted actions, such as transferring money or changing account settings, without their knowledge or consent. The attacker takes advantage of the user’s active session with the application to carry out these actions, often by tricking the user into clicking a link or submitting a form on a malicious website.
To protect your application from CSRF attacks, including a unique CSRF token in every form submission is essential. This token is a secret code known only to the server and the legitimate user. When a form is submitted, the server checks to see if the token sent by the user matches the one stored on the server, ensuring the request is legitimate.
Here’s how to implement CSRF protection in PHP:
- Generate a CSRF token: This token should be unique for each session and each form submission. You can generate a token using PHP’s random_bytes() function and store it in the user’s session.
// Generate a CSRF token and store it in the session
$_SESSION[‘csrf_token’] = bin2hex(random_bytes(32));
- Include the token in the form: When creating forms that perform sensitive actions, include the CSRF token as a hidden input field. This ensures that the form includes the token when submitted.
<form method=”POST”>
<input type=”hidden” name=”csrf_token” value=”<?= $_SESSION[‘csrf_token’] ?>”>
<input type=”submit” value=”Submit”>
</form>
- Verify the token on the server side: When the form is submitted, you need to compare the CSRF token sent by the user (via the hidden form field) with the token stored in the session. If the tokens do not match, the request is likely a CSRF attack, and you should reject it.
// Check if the CSRF token is valid
if ($_POST[‘csrf_token’] !== $_SESSION[‘csrf_token’]) {
// Token mismatch: Possible CSRF attack
die(‘Invalid CSRF token’);
}
// Process the form data if the token is valid
Following these steps can effectively prevent CSRF attacks in your PHP application. The CSRF token ensures that any request made by the user is legitimate and not a malicious action initiated by an attacker. This simple measure improves the security of your web application.
Session Hijacking
Session hijacking is an attack where an attacker steals a user’s session cookie, allowing them to impersonate the user and gain unauthorized access to their account. This poses a serious threat to web applications because it can expose sensitive information and enable attackers to perform actions as if they were legitimate users.
To protect your application from session hijacking, here are several essential security measures you can implement:
- Bind Sessions to the User’s IP Address: One way to make session hijacking more difficult is by tying the session to the user’s IP address. This means that even if an attacker steals the session cookie, they won’t be able to use it unless they are on the same network or device as the legitimate user. However, remember that this may cause problems for users with dynamic IP addresses.
- Session Timeout Mechanism: A session timeout is another effective way to limit an attacker’s time to hijack a session. After a period of inactivity, the session should automatically expire, requiring the user to log in again. This reduces the risk of unauthorized access.
- Regenerate Session IDs: To prevent session fixation attacks (where an attacker sets a known session ID before the user logs in), you should regenerate the session ID after login or at regular intervals. In PHP, you can use the session_regenerate_id() function to do this:
// Regenerate session ID to prevent session fixation attacks
session_regenerate_id(true);
This function creates a new session ID, invalidating any previously issued IDs.
- Secure Session Cookies: To protect session cookies from being easily accessed by attackers, you should store them securely by setting the HttpOnly and Secure flags:
- HttpOnly Flag: This flag makes the session cookie inaccessible to JavaScript, helping prevent attacks like cross-site scripting (XSS), where an attacker tries to steal cookies through malicious scripts.
- Secure Flag: This flag ensures that the session cookie is only sent over secure HTTPS connections, protecting it from being intercepted during transmission.
Here’s how you can set these flags in PHP:
// Ensure session cookies are secure
ini_set(‘session.cookie_httponly’, 1); // Make cookie accessible only to HTTP requests
ini_set(‘session.cookie_secure’, 1); // Only send cookies over HTTPS
Following these best practices can significantly reduce the risk of session hijacking and keep your PHP application secure. Remember, session security is crucial for protecting user data and maintaining trust in your web application.
Secure File Uploads
If not handled properly, file uploads can pose a security risk. Attackers may try to upload malicious files, such as scripts or executable files, to exploit weaknesses in your application. This is particularly dangerous if user-uploaded files are not validated correctly. Implementing strong security measures to reduce this risk and ensure uploaded files are safe and won’t cause harm is essential.
Here are some essential steps to secure file uploads:
- Validate the MIME Type: The MIME type tells you the file’s content type. Always validate the MIME type to ensure the file matches the expected type (e.g., an image or document). PHP’s finfo class can check the MIME type based on the file’s content.
- Check the File Extension: Besides validating the MIME type, check the file extension to add another layer of protection. Ensure the extension matches the expected file type (e.g., .jpg or .png for images).
- Store Files in a Secure Location: Always store uploaded files outside the web root directory. This prevents direct access to the files through the web server. For example, avoid storing user-uploaded files in a publicly accessible folder like uploads/. Instead, store them in a non-web-accessible directory and use server-side scripts to serve the files when needed.
- Process Only Safe File Types: To ensure only certain types of files (e.g., images or documents) are allowed, validate the MIME type against a list of allowed types. For example, check if the file is an image by validating its MIME type before processing it. Here’s how you can do it in PHP:
// Get the MIME type of the uploaded file
$finfo = new finfo(FILEINFO_MIME_TYPE);
$fileContents = file_get_contents($_FILES[‘file’][‘tmp_name’]);
$mimeType = $finfo->buffer($fileContents);
// Check if the MIME type is an allowed image type (JPEG or PNG)
if (in_array($mimeType, [‘image/jpeg’, ‘image/png’])) {
// Process the file (e.g., save it to a secure location)
} else {
// Reject the file if it’s not an allowed type
echo “Invalid file type. Only JPEG and PNG images are allowed.”;
}
In this example:
- We first use the finfo function to get the MIME type of the uploaded file.
- Then, we check if the MIME type is in the list of allowed types (e.g., image/jpeg or image/png).
- If the file type is valid, it can be processed further (e.g., saved to a secure location).
- If the file type is invalid, it’s rejected, and an error message is shown.
Following these steps can significantly reduce the risk of uploading malicious files and ensure your application handles file uploads securely. Never blindly trust user input; validate and sanitize files at every step.
Use SSL for HTTPS
SSL certificates (Secure Sockets Layer) protect sensitive information exchanged between a user’s browser and a server. They encrypt data, ensuring that anything transmitted, such as passwords, payment details, and personal information, stays secure and cannot be intercepted by attackers.
When a website uses HTTPS (HyperText Transfer Protocol Secure), the communication between the user’s browser and the server is encrypted with SSL/TLS. This prevents unauthorized parties, such as hackers, from listening to the data.
To improve security, it is essential to ensure all traffic to your site is encrypted with HTTPS. This means users should always access the site through a secure connection, even if they try to visit the HTTP version. If your website supports both HTTP and HTTPS, attackers could intercept data on the HTTP version, making it vulnerable.
To ensure HTTPS is used throughout your website, you can automatically redirect users from the HTTP version to the HTTPS version. Here’s a simple way to do this using PHP:
// Check if the request is not secure (i.e., not using HTTPS)
if ($_SERVER[‘HTTPS’] != ‘on’) {
// Redirect to the HTTPS version of the current page
header(‘Location: https://’ . $_SERVER[‘HTTP_HOST’] . $_SERVER[‘REQUEST_URI’]);
exit(); // Stop further script execution to ensure the redirect happens immediately
}
Here’s how this works:
- The code checks whether the current request uses HTTPS by checking $_SERVER[‘HTTPS’].
- If the value is not ‘on’ (which means it’s not using HTTPS), the user is redirected to the HTTPS version of the same URL using the header(‘Location: https://…’).
- $_SERVER[‘HTTP_HOST’] contains the domain name, and $_SERVER[‘REQUEST_URI’] contains the requested URL’s full path and query string.
- The exit() function ensures the script stops after redirecting, preventing further code execution.
By using this redirect, you ensure that all users are directed to a secure, encrypted version of your site, protecting sensitive information from potential attackers. It’s a vital step in securing your website and maintaining user trust.
Cloud Deployment and Server Security
Deploying PHP applications on cloud hosting platforms like AWS (Amazon Web Services) and DigitalOcean offers substantial security benefits. These cloud providers offer advanced security features that help protect your application from various threats, ensuring the safety of your data and resources.
For example, cloud hosting platforms often include built-in DDoS (Distributed Denial of Service) protection. DDoS attacks attempt to overwhelm your server with too much traffic, causing it to slow down or crash. Cloud providers use advanced tools and algorithms to detect and stop these attacks before they can damage your server or website.
Besides DDoS protection, cloud services offer firewalls, which act as barriers between your application and potential attackers. Firewalls monitor incoming and outgoing traffic, allowing only trusted traffic to reach your server and blocking harmful attempts.
Key Security Tips for Cloud Hosting:
- Configure Your Server’s Firewall: A well-configured firewall controls who can access your server. It blocks unauthorized traffic and protects your server from attacks. You can set rules to allow traffic only from trusted sources and block others.
- Restrict Database Access: Databases are vital to your application, so securing them is essential. Limit access by whitelisting specific IP addresses, meaning only trusted servers or users with approved IP addresses can connect to your database. This helps prevent unauthorized access by hackers or malicious actors.
- Use Additional Security Layers: Cloud providers often offer extra security features, such as virtual private networks (VPNs) and data storage and transmission encryption. Enabling these features can further strengthen the security of your PHP application.
- Keep Your Software Updated: Even with cloud providers’ security features, it’s still important to regularly update your server and application software. Many vulnerabilities come from outdated software, so quickly applying security patches and updates helps reduce risks.
By using the security tools offered by cloud platforms and configuring them correctly, you can significantly improve the security of your PHP applications. This ensures your application stays safe and reliable, even in the face of potential cyber threats.
Common Vulnerabilities in PHP-based CMS
Popular content management systems (CMS) like WordPress, Joomla, and Magento are often targeted because they are widely used. Each CMS has its security challenges:
- WordPress: It is frequently targeted because it uses many plugins and themes, which can create vulnerabilities.
- Joomla: Although it is more secure by default, it still faces risks from outdated extensions and weak passwords.
- Magento: As an e-commerce platform, Magento has unique challenges in securing payment gateways and protecting customer data.
To secure these systems, ensure all plugins, themes, and extensions are up to date and implement strong access control methods, such as multi-factor authentication (MFA).
Conclusion
PHP security is essential to protect web applications and user data from cyberattacks. Developers can reduce common vulnerabilities by following best practices such as regularly updating PHP versions, using prepared statements to prevent SQL injection, employing CSRF tokens, and securing file uploads. While no system is entirely immune to threats, adopting strong security measures greatly lowers the risk of breaches and ensures the safety of your PHP web applications.
As the PHP ecosystem evolves, staying informed about security updates and vulnerabilities is crucial to securing your applications. Developers can build safe and reliable web applications by consistently applying security best practices and using modern PHP frameworks.